Customizing Guide to Godot How to Hard Edit the Binding for UI_Left
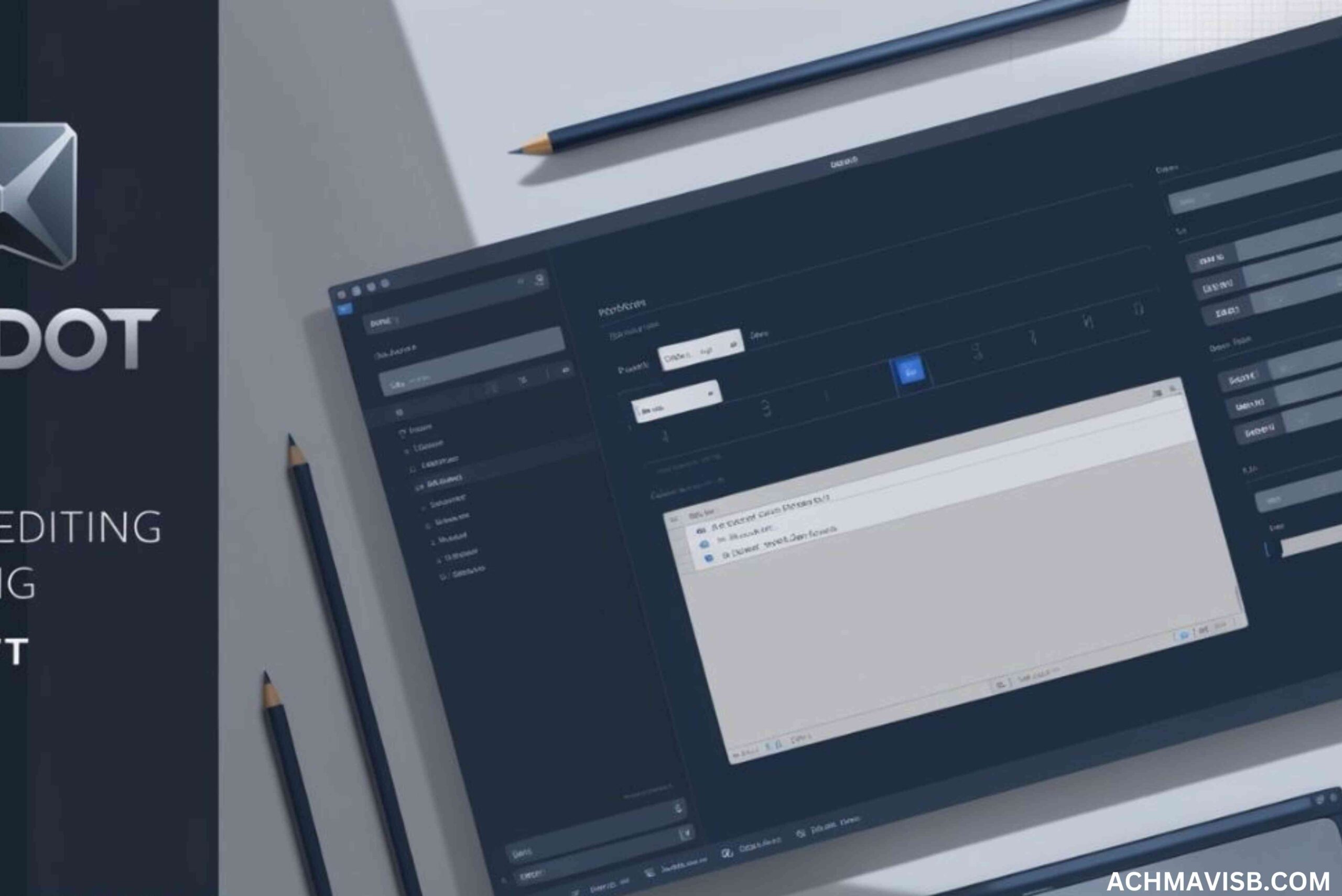
If you’ve ever worked with Godot, the open-source game engine, you’ve likely encountered its input system. But what happens when you need to customize something deeper—like editing the binding for a specific action such as ui_left
? In this article, we’ll break it all down for you, step by step, so you can tweak your controls like a pro.
Table of Contents
Toggle1. Introduction to Godot How To Hard Edit The Binding For ui_left
Have you ever played a game and thought, “This control doesn’t feel right?” Input bindings are what make your keyboard, mouse, or gamepad actions translate into in-game movements. In Godot, these bindings can be customized for better control and accessibility.
2. What is ui_left in Godot?
godot how to hard edit the binding for ui_left is one of Godot’s default input actions. It’s typically bound to the left arrow key, but you can assign it to other keys or buttons depending on your game’s needs. Imagine it as the steering wheel of your car—it’s crucial to get it just right!
3. Why Customize Input Bindings?
Sometimes the default settings don’t work for everyone. Maybe you’re creating a game with unique controls, or perhaps you want to offer accessibility options for players. Either way, customizing bindings is essential.
4. Understanding Godot’s Input Map
The Input Map in Godot is where all your actions and their associated keys are stored. Think of it as a dictionary where the keys are input actions like ui_left
, and the values are the actual buttons or keys that trigger them.
Key Features of the Input Map:
Supports multiple bindings per action
Works with keyboards, gamepads, and other devices
Easy to extend for custom actions
5. How to Access Input Settings
To access the Input Map in Godot:
Open your project in the Godot editor.
Go to Project > Project Settings.
Navigate to the Input Map tab.
Here, you can view and modify existing bindings or create new ones.
6. Editing Bindings via UI
The easiest way to change a binding is through the Input Map UI:
Select the action (e.g.,
ui_left
).Click the “Add” button to assign a new key or button.
Remove unwanted bindings by clicking the trash icon next to them.
But what if you need more control or automation? That’s where hard editing comes in.
7. Hard Editing Input Bindings in Code
Hard editing refers to modifying input bindings directly in code. This approach is perfect if you want to:
Dynamically change bindings at runtime.
Save custom bindings for individual players.
How Does It Work?
You’ll use Godot’s InputMap
class to programmatically manage bindings. Here’s how:
InputMap.action_erase_events("ui_left")
InputMap.action_add_event("ui_left", InputEventKey.new().set_scancode(KEY_A))
This snippet clears existing bindings for ui_left
and assigns the A
key to it.
8. Example: Rebinding ui_left
Let’s walk through an example where we rebind ui_left
to the A
key:
Step-by-Step Guide:
Open your Godot script editor.
Add the following code to your script:
extends Node
func _ready():
# Remove existing bindings
InputMap.action_erase_events("ui_left")
# Create a new input event
var new_event = InputEventKey.new()
new_event.scancode = KEY_A
# Add the new binding
InputMap.action_add_event("ui_left", new_event)
print("Rebound ui_left to A key!")
Run your scene to apply the changes.
9. Testing Your Changes
Testing is a crucial step. Ensure your changes work as intended by:
Running your game.
Using debugging tools to monitor input.
Checking for conflicts with other bindings.
10. Common Issues and Fixes
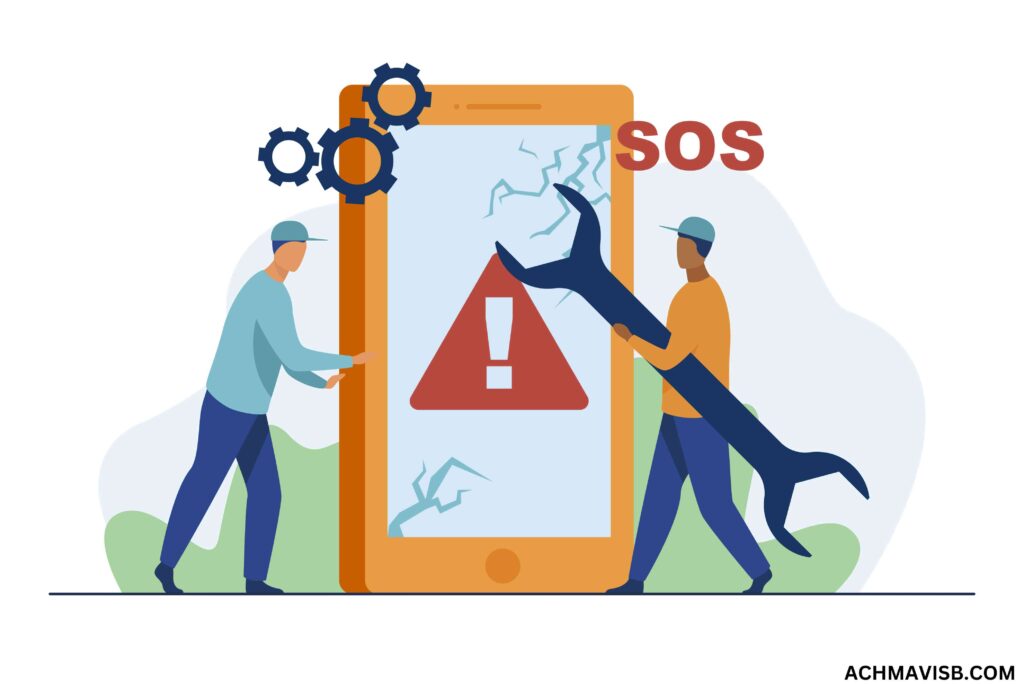
Issue 1: Binding Doesn’t Work
Fix: Ensure the action name matches exactly.
Issue 2: Conflicting Bindings
Fix: Check for overlapping keys and resolve conflicts.
Issue 3: Custom Binding Not Saved
Fix: Implement a save system for bindings (covered below).
11. Saving and Loading Custom Bindings
To save custom bindings, you’ll need to write them to a file. Here’s an example:
func save_bindings():
var file = File.new()
file.open("user://bindings.cfg", File.WRITE)
file.store_var(InputMap.get_action_list())
file.close()
func load_bindings():
var file = File.new()
if file.file_exists("user://bindings.cfg"):
file.open("user://bindings.cfg", File.READ)
var bindings = file.get_var()
InputMap.load(bindings)
file.close()
12. Debugging Input in Godot
Use tools like the Input Debugger to monitor active inputs. It’s like having a magnifying glass to pinpoint what’s happening under the hood.
13. Best Practices for Input Customization
Always provide default bindings as a fallback.
Allow players to customize controls in-game.
Test on multiple devices to ensure compatibility.
14. Advanced Tips for Input Handling
Tip 1: Use Input Groups
Group similar actions (e.g., movement) for better organization.
Tip 2: Handle Multiple Devices
Support keyboards, controllers, and touchscreens seamlessly.
Tip 3: Dynamic Rebinding
Use scripts to allow players to rebind keys during gameplay.
15. Conclusion and FAQs
Customizing input bindings, like editing, is a vital skill for any Godot developer. Whether you’re tweaking controls for accessibility or crafting a unique gameplay experience, the steps outlined here will set you up for success.
FAQs
- Can I rebind actions without writing code?
Yes, you can use the Input Map UI in the Project Settings.
- How do I support multiple input devices?
Use Godot’s input handling to assign bindings for keyboards, gamepads, and touchscreens.
- What’s the best way to save custom bindings?
Save them to a configuration file using Godot’s File class.
- Can I detect input conflicts programmatically?
Yes, by checking for overlapping bindings in the Input Map.
- Is there a way to reset bindings to default?
Yes, maintain a set of default bindings and reload them when needed.